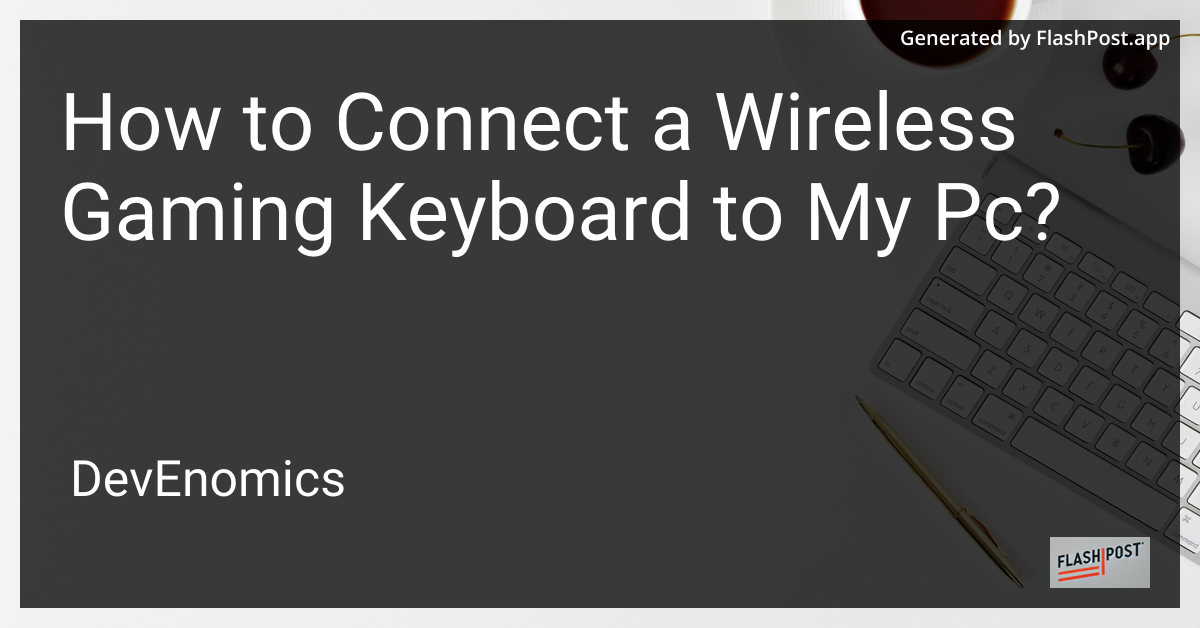
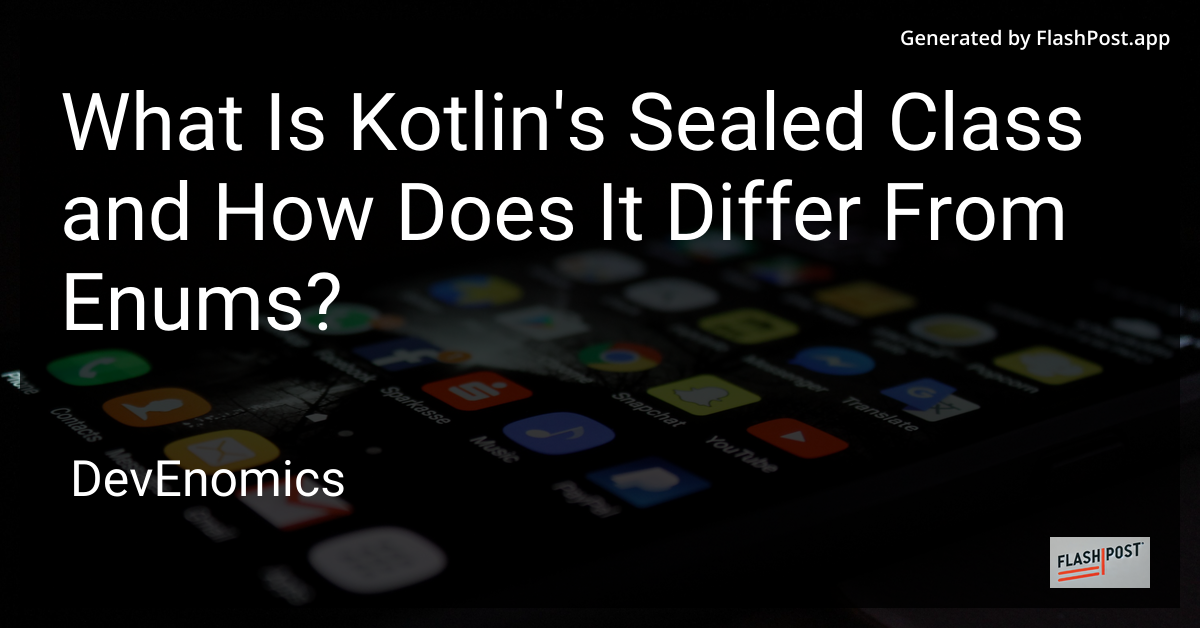
What Is Kotlin's Sealed Class and How Does It Differ From Enums?
Kotlin, a modern, statically typed programming language on the JVM, has been steadily gaining popularity due to its expressive syntax and powerful feature set. Among its compelling features are sealed classes and enums, which offer unique ways to represent restricted hierarchies of types. Let’s dive into what Kotlin’s sealed classes are, how they differ from enums, and why understanding these differences can lead to better software design.
What Are Sealed Classes in Kotlin?
Sealed classes in Kotlin are a special type of class that allow developers to represent restricted class hierarchies. You can think of them as an advanced version of enums. Sealed classes let you create a limited set of subclasses, which means all possible subclasses are known at compile time. This feature ensures exhaustive when
expressions, making your code more reliable and easier to maintain.
Characteristics of Sealed Classes:
- Restrictive Subclassing: Only subclasses defined in the same file can inherit from a sealed class.
- Exhaustive
when
Expressions: Kotlin enforces exhaustivewhen
expressions on sealed classes, ensuring that you handle all possible subclasses. - Abstract by Default: Sealed classes cannot be instantiated directly. They must be subclassed.
- State Representation: Each subclass of a sealed class can hold additional data, offering more flexibility in representing state.
To see more about how Kotlin simplifies development in comparison to Java, check out Kotlin over Java Advantages.
How Enums Differ From Sealed Classes
Kotlin’s Enum
class is a type used to define a collection of constants. They are perfect for cases where you need a simple set of distinct values. Unlike sealed classes, enums are instances of a single type and do not allow for additional data or state.
Characteristics of Enums:
- Single Instance per Constant: Each enum constant is an instance of the enum class.
- Predefined Instances: Enum constants are predefined and implicitly public static final.
- Simple State Representation: No additional state or subclassing possible.
Enums are ideal for when you need a fixed set of related constants without the complexity or need for state representation that sealed classes offer.
For techniques to convert enums in Kotlin, refer to Enum Conversion Techniques in Kotlin.
When to Use Sealed Classes vs Enums
Here are some guidelines to decide when to use sealed classes over enums:
-
Use Sealed Classes when you need to represent a hierarchy that requires different states or behavior for each type. They are perfect for complex scenarios such as representing results from network operations with states like
Success
,Error
, andLoading
. -
Use Enums when you have a limited set of constant values and the associated logic doesn’t require additional state beyond what the constants provide.
Conclusion
Kotlin’s sealed classes and enums both provide powerful ways to handle type hierarchies. By understanding the differences between them and knowing when to use each, you can write more expressive, maintainable, and bug-free code. Whether dealing with network responses, UI states, or configuration options, make the most of these tools to enhance your Kotlin applications. For dynamically parsing data using Kotlin, consider reading how to parse JSON in Kotlin.
By leveraging Kotlin’s robust features like sealed classes, developers can simplify complex type management in their applications, leading to code that is not just functional, but highly readable and maintainable.