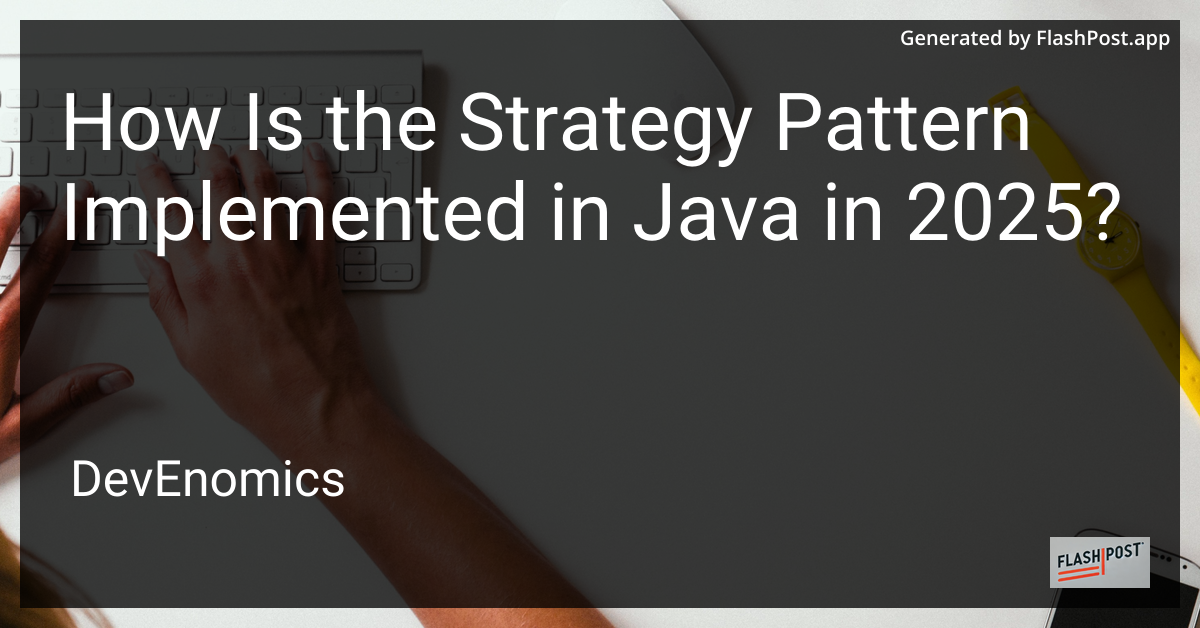
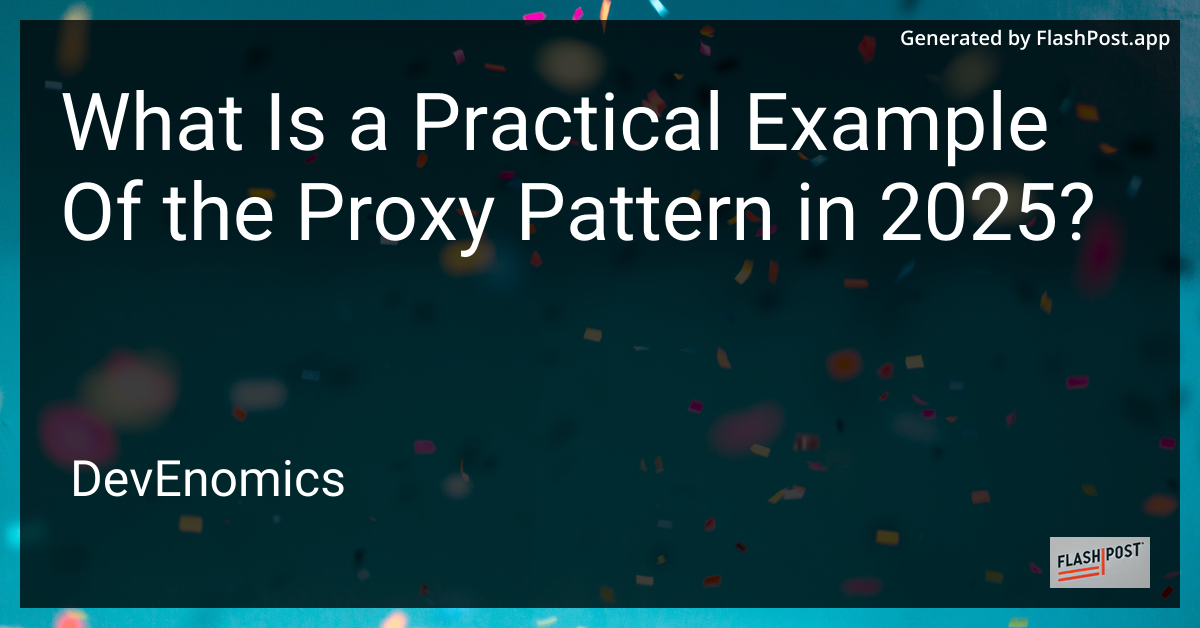
What Is a Practical Example Of the Proxy Pattern in 2025?
The proxy pattern is a structural design pattern that provides a surrogate or placeholder for another object to control access to it. By introducing an additional layer of abstraction, this pattern proves incredibly useful in various software development scenarios. As we step into 2025, let’s explore how the proxy pattern can be practically applied in a modern context.
What is the Proxy Pattern?
The proxy pattern enables a controlled mechanism of access to an object, often referred to as the real subject. A proxy object has the same interface as the real subject, allowing clients to use them interchangeably. This pattern is instrumental in scenarios where you need to add functionality to an object and control its access while ensuring efficiency and security.
Practical Example in 2025: Cloud-Based Resource Management
Imagine a cloud-based application where multiple services request access to a particular resource, such as data stored in a remote server or computational resources from a cloud provider.
Scenario
Consider a distributed application that processes a large amount of data. Directly accessing the data from individual nodes can result in performance bottlenecks and security vulnerabilities due to concurrent access attempts. This is where the proxy pattern shines as a solution.
Implementation
In our 2025 application, we can implement a DataResourceProxy to manage the requests to these cloud resources. This proxy will:
-
Control Access: Monitor and regulate which clients can access the resource at what times. This ensures that no single client over-consumes the resource, leading to fair use among all requesting services.
-
Cache Responses: To optimize performance, the proxy can cache data responses so that repeated requests for the same data don’t hit the primary data store repeatedly, thereby significantly reducing latency.
-
Security and Authentication: By sitting between the client and the resource, the proxy can enforce security policies, authenticating clients before forwarding requests to the actual resource.
-
Logging and Monitoring: The proxy can log usage statistics and monitor performance, giving insights into how resources are utilized and helping optimize future resource allocations.
class CloudResourceProxy:
def __init__(self, resource):
self._resource = resource
self._cache = {}
self._access_log = []
def request_data(self, client_id, data_id):
if not self._authenticate(client_id):
raise PermissionError("Access Denied")
if data_id in self._cache:
return self._cache[data_id]
data = self._resource.get_data(data_id)
self._cache[data_id] = data
self._log_access(client_id, data_id)
return data
def _authenticate(self, client_id):
# Implement authentication logic
return True
def _log_access(self, client_id, data_id):
self._access_log.append((client_id, data_id))
Here, the CloudResourceProxy
class controls access while also handling authentication, caching, and logging. It ensures that real subject functionalities are not exposed directly to the client, thereby maintaining abstraction and security.
Conclusion
The proxy pattern continues to evolve as a significant design principle in 2025, reflecting modern challenges in resource management and security. Its ability to provide controlled access and enhance performance makes it indispensable in cloud-based applications and beyond.
For further reading on design patterns and their applications, consider exploring the following resources:
- Check out the best deals on design patterns literature to deepen your understanding of this and more patterns.
- Explore how similar patterns like MVC are implemented in Tkinter design patterns.
- For a broader perspective, see implementing design patterns in different languages like Rust.
By embracing such patterns, developers can create robust and scalable systems ready to tackle the complexities of modern software development.