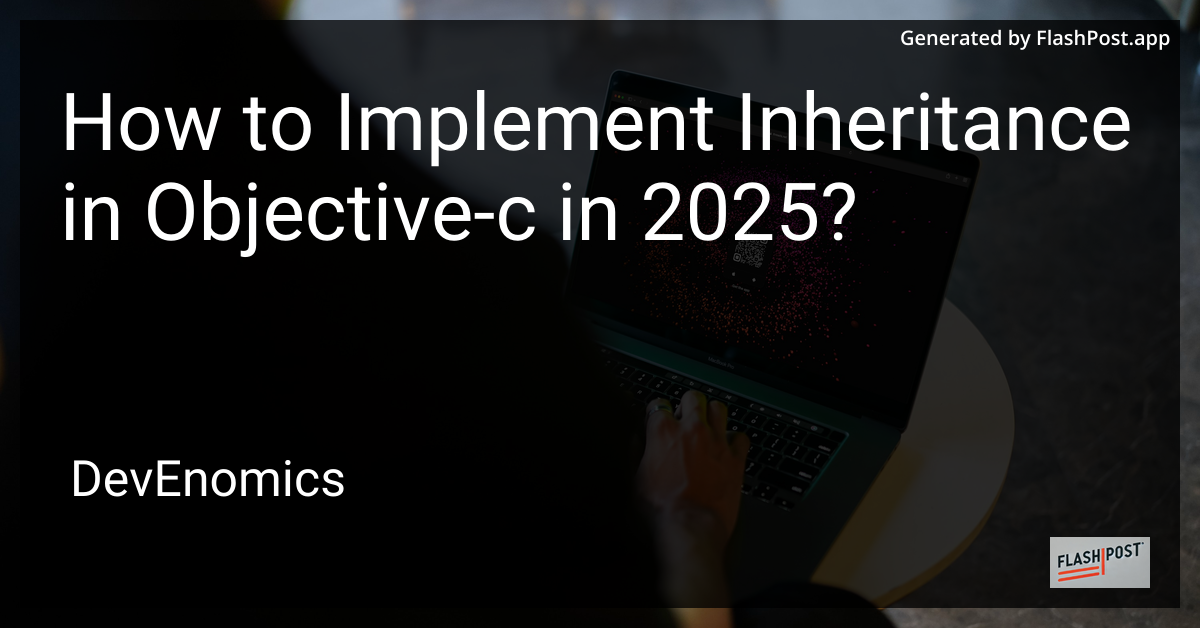
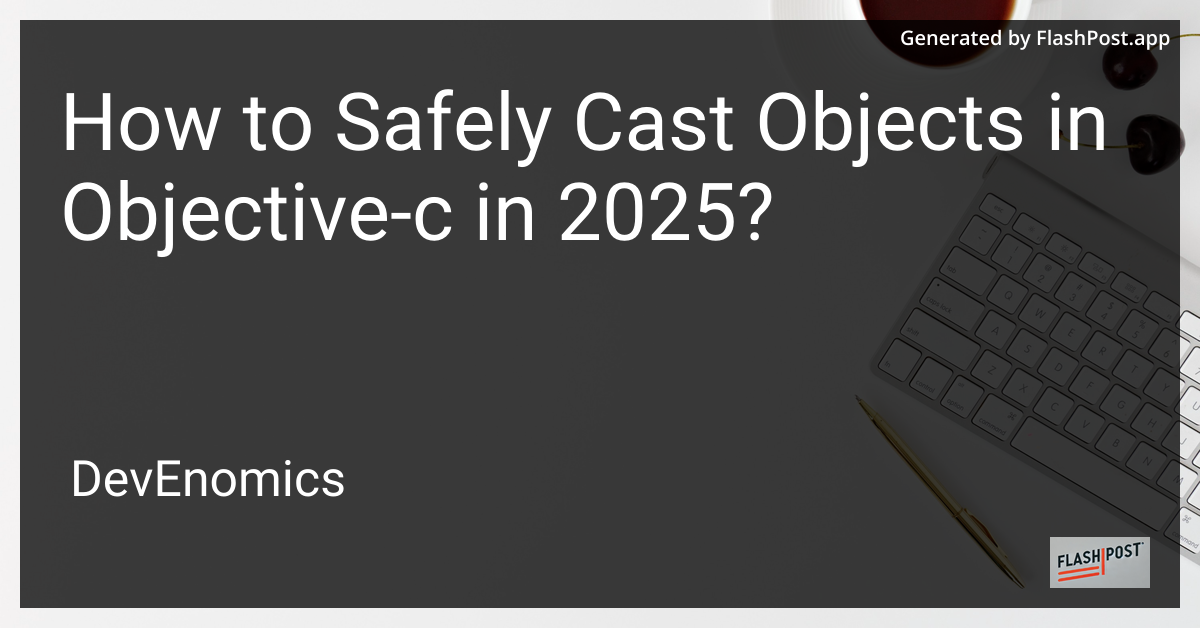
How to Safely Cast Objects in Objective-c in 2025?
Casting objects safely in Objective-C remains an essential practice for developers, ensuring that your applications are robust and free from unexpected crashes. As we move into 2025, understanding best practices for object casting can greatly enhance code reliability and maintainability. This article provides insights into methods for safely casting objects, while also adhering to modern Objective-C practices.
Understanding Object Casting in Objective-C
Object casting involves converting a pointer of one type into another. In Objective-C, this is often necessary due to the dynamic nature of the language. Improper casting can lead to runtime errors, which are notoriously difficult to debug. Safe casting reduces such risks, ensuring that your application handles objects correctly at all times.
Best Practices for Safe Object Casting
1. Use isKindOfClass
Method
Before performing a cast, you must check whether the object is of a compatible class type. The isKindOfClass
method allows you to determine if an object is an instance of a particular class or a subclass:
if ([anObject isKindOfClass:[NSString class]]) {
NSString *string = (NSString *)anObject;
// Safe to use string here
}
2. Employ respondsToSelector
Another layer of safety is checking if an object can respond to a specific method, using respondsToSelector
:
if ([anObject respondsToSelector:@selector(lowercaseString)]) {
NSString *string = (NSString *)anObject;
NSString *lowercaseString = [string lowercaseString];
// Perform operations safely
}
3. Use Lightweight Generics
Objective-C introduced lightweight generics to improve type safety. When working with collections, specify the type of objects they contain, which helps the compiler catch type mismatches at compile-time:
NSArray<NSString *> *stringArray = @[@"one", @"two", @"three"];
NSString *firstString = stringArray.firstObject;
// Compiler ensures firstObject is a NSString
4. Advocate for Defensive Programming
Even with checks, it’s wise to program defensively. Implement error handling to manage unexpected object types gracefully:
if (![anObject isKindOfClass:[NSString class]]) {
NSLog(@"Error: Expected a NSString but received %@", NSStringFromClass([anObject class]));
} else {
NSString *string = (NSString *)anObject;
// Safe to use string here
}
Additional Resources
- Learn more about documenting Objective-C blocks using Doxygen.
- Set up your Objective-C projects for continuous inspection with SonarQube.
- Explore the latest deals on top Objective-C programming books at Top Deals Net.
Conclusion
While Objective-C allows for dynamic object handling, it’s critical to perform safe casting to ensure application stability. By utilizing these methods — such as isKindOfClass
checks, respondsToSelector
verification, and leveraging lightweight generics — developers can minimize runtime errors and maintain clean, efficient code in 2025 and beyond.
Remember, embracing these practices not only enhances your app’s resilience but also streamlines the development process, allowing you to focus on creating exceptional iOS applications.