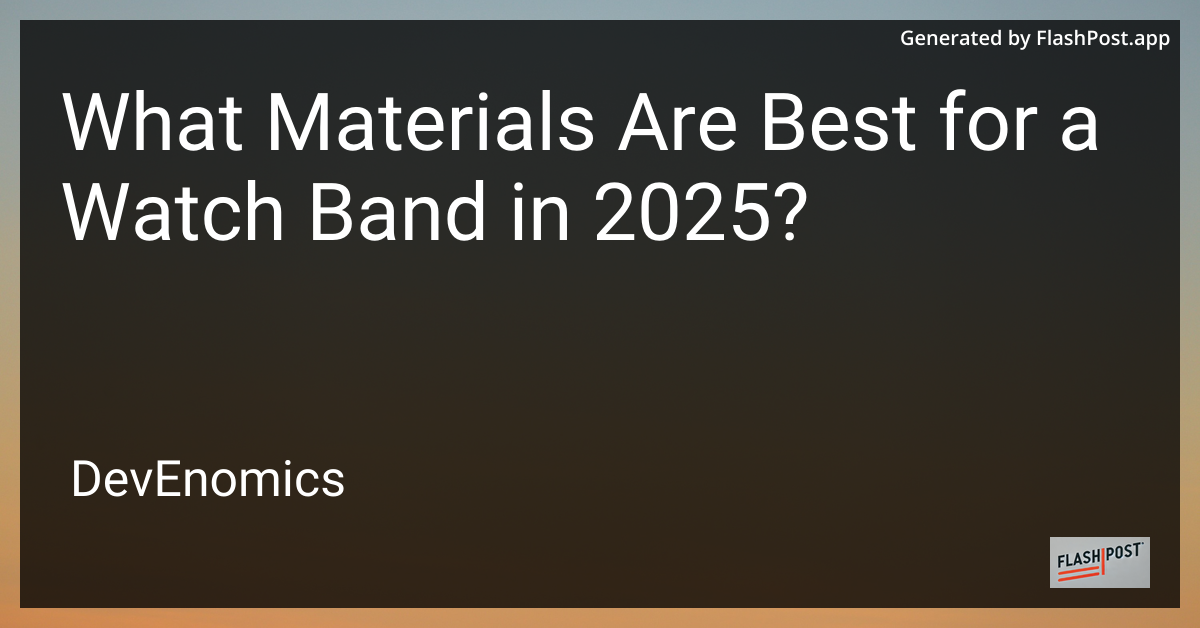
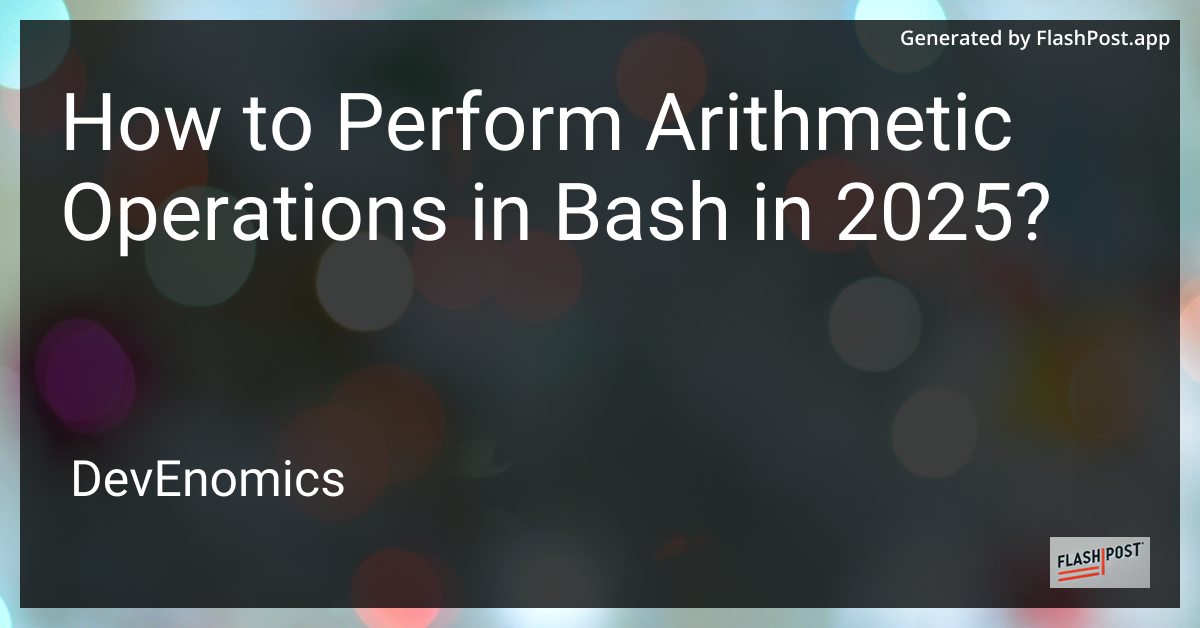
How to Perform Arithmetic Operations in Bash in 2025?
As programming continues to evolve, Bash scripting remains a fundamental skill for anyone involved in system administration or automation tasks. One of the essential aspects of Bash scripting is performing arithmetic operations. By 2025, Bash scripts have become even more robust, leveraging improvements in computational logic and enhanced syntax. This article will guide you through performing arithmetic operations in Bash, focusing on the best practices and updates that have emerged.
Basic Arithmetic Operations in Bash
Bash uses a variety of tools to perform arithmetic operations. The most common method involves using the (( ))
syntax and the let
command. In addition, expr and bc are also used for more complex calculations.
Using Double Parentheses (( ))
The (( ))
syntax is straightforward and allows you to perform arithmetic operations directly. It supports standard arithmetic operators such as +
, -
, *
, /
, and %
.
Example:
#!/bin/bash
a=10
b=5
(( sum = a + b ))
echo "Sum: $sum"
(( diff = a - b ))
echo "Difference: $diff"
(( product = a * b ))
echo "Product: $product"
(( quotient = a / b ))
echo "Quotient: $quotient"
(( modulus = a % b ))
echo "Modulus: $modulus"
Using the let
Command
The let
command is another way to perform arithmetic in Bash scripts. It is similar to using double parentheses but requires each operation to be executed as a separate command.
Example:
#!/bin/bash
x=20
y=4
let sum=x+y
let diff=x-y
let product=x*y
let quotient=x/y
let modulus=x%y
echo "Sum: $sum"
echo "Difference: $diff"
echo "Product: $product"
echo "Quotient: $quotient"
echo "Modulus: $modulus"
Using expr
expr
is a command utilized for evaluating expressions. It outputs the result on the standard output.
Example:
#!/bin/bash
sum=$(expr 15 + 5)
echo "Sum: $sum"
diff=$(expr 20 - 5)
echo "Difference: $diff"
product=$(expr 6 \* 7)
echo "Product: $product"
quotient=$(expr 36 / 4)
echo "Quotient: $quotient"
Using bc
for Complex Calculations
bc
stands for “Basic Calculator” and is used for more complex arithmetic operations, particularly those involving floating-point calculations.
Example:
#!/bin/bash
echo "4.5 + 5.2" | bc
echo "scale=2; 15.5 / 2" | bc
Conclusion
Performing arithmetic operations in Bash has never been easier, thanks to the variety of tools available. By 2025, these methods continue to be reliable and efficient for any script-based arithmetic calculations. Using these techniques ensures that your Bash scripts are both powerful and maintainable.
For further learning on Bash scripting, you may find the following resources useful:
- Bash Scripting Tutorial: A comprehensive guide to mastering Bash scripting.
- Match in Bash: A resourceful forum for Bash scripting discussions.
- Bash Script Tips: Enhance your Bash scripting skills with expert advice.
With the continued relevance and utility of Bash, mastering arithmetic operations will significantly improve your scripting efficiency and effectiveness. Happy scripting!