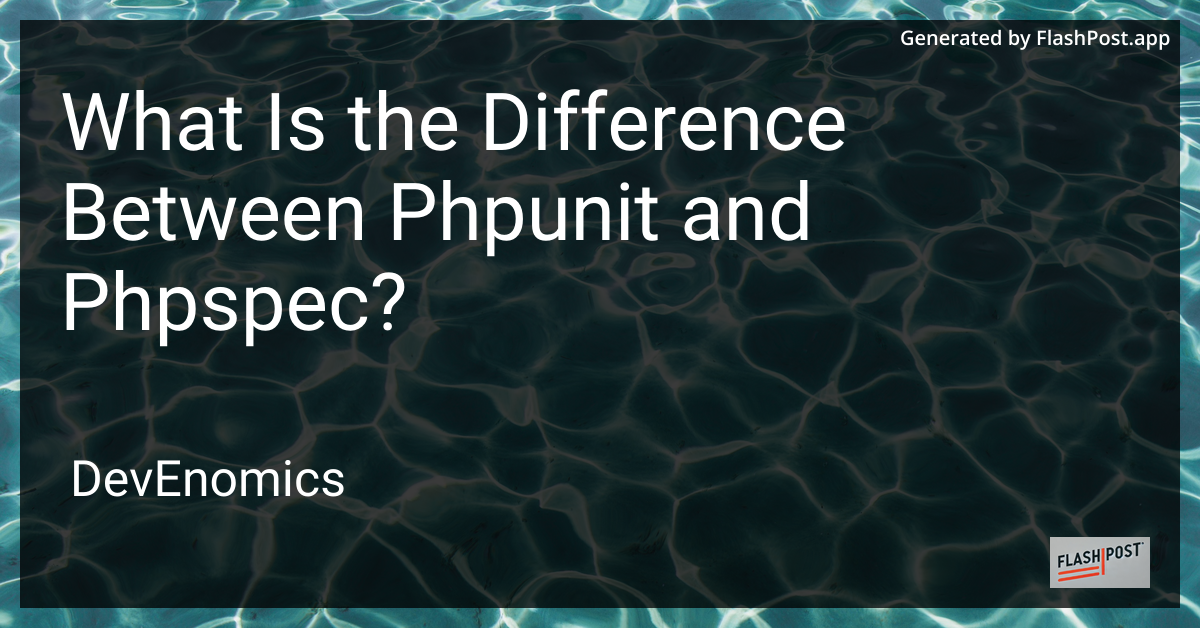
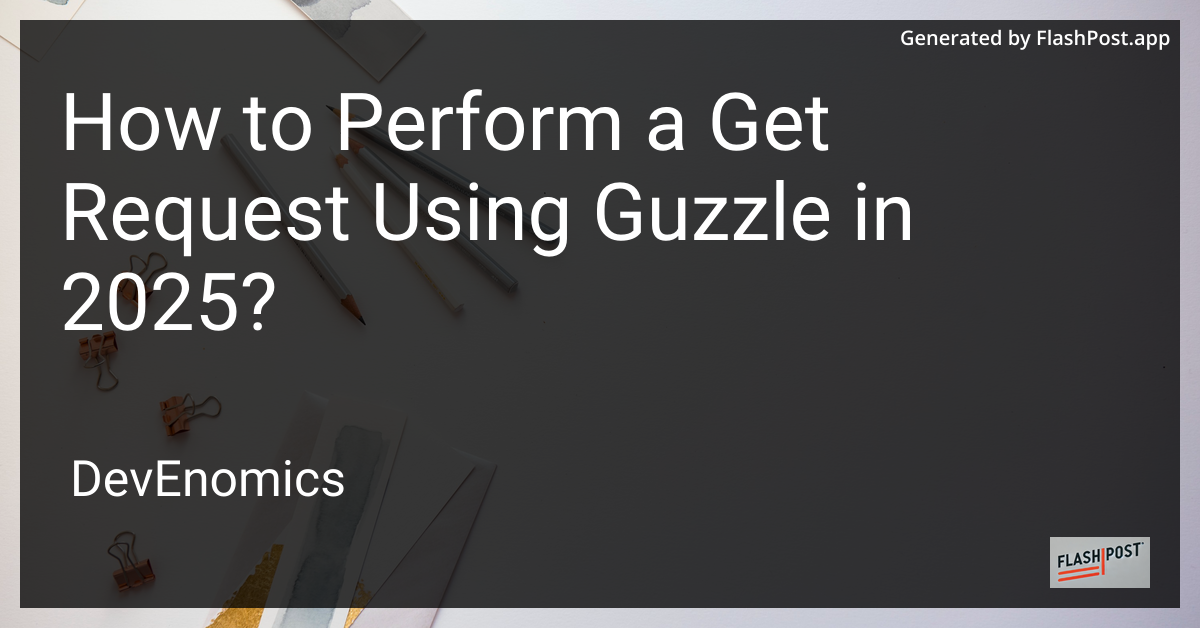
How to Perform a Get Request Using Guzzle in 2025?
title: How to Perform a GET Request Using Guzzle in 2025 description: Learn how to efficiently execute a GET request with Guzzle HTTP client in 2025 to boost your web application’s performance and integration capabilities. keywords: GET request, Guzzle, HTTP client, PHP, Laravel, web development
As web development continues to evolve, the need for efficient and reliable HTTP clients remains vital for seamless application integrations. Guzzle, a PHP HTTP client, remains a top choice for developers seeking to interact with RESTful APIs. In this article, we will explore how to perform a GET request using Guzzle in 2025, leveraging the latest best practices and features.
What is Guzzle?
Guzzle is a popular HTTP client for PHP that provides a simple and elegant interface to send HTTP requests and handle their responses. It’s known for its ease of use, powerful features, and flexible, extensible architecture.
Prerequisites
Before proceeding, ensure you have Guzzle installed in your PHP project. If not, you can install it via Composer:
composer require guzzlehttp/guzzle
Ensure you have PHP 8.0 or higher, as support for older PHP versions diminishes over the years.
Step-by-Step Guide to Perform a GET Request Using Guzzle
-
Initialize Guzzle Client
Begin by creating an instance of the Guzzle HTTP client:
use GuzzleHttp\Client; $client = new Client();
-
Configure the Request
Set up the necessary parameters for the GET request. This might include query parameters, headers, and other configurations:
$url = 'https://api.example.com/data'; $options = [ 'headers' => [ 'Accept' => 'application/json', 'User-Agent' => 'my-app/1.0' ], 'query' => ['key1' => 'value1', 'key2' => 'value2'] ];
-
Send the GET Request
Use the
get
method of the client instance to send the request:try { $response = $client->get($url, $options); // Getting the response body $body = $response->getBody()->getContents(); $data = json_decode($body, true); // Displaying the response data print_r($data); } catch (\Exception $e) { // Handle exceptions echo 'Request failed: ' . $e->getMessage(); }
-
Handle the Response
Successfully sending a request will give you a
Response
object which you can use to handle the data returned by the API.if ($response->getStatusCode() == 200) { // Successful response echo "Success! Data received."; } else { echo "Failed to retrieve data."; }
Enhancing Security
While Guzzle is a powerful tool, it’s important to consider SSL verification for secure data transmission. To learn more about handling SSL verification in Guzzle, you may read these articles:
Conclusion
With the continued growth of web applications and APIs, using Guzzle to perform GET requests in PHP projects remains a valuable skill. Knowing how to configure requests and handle responses efficiently will ensure your web applications are fast, reliable, and secure. Stay updated with Guzzle’s latest features to enhance your development workflows in 2025.
By integrating the steps outlined above, you can adeptly handle GET requests in your PHP applications using Guzzle. This client library remains crucial for robust API interactions and reliable data exchanges, ensuring your development in 2025 is at its most efficient.