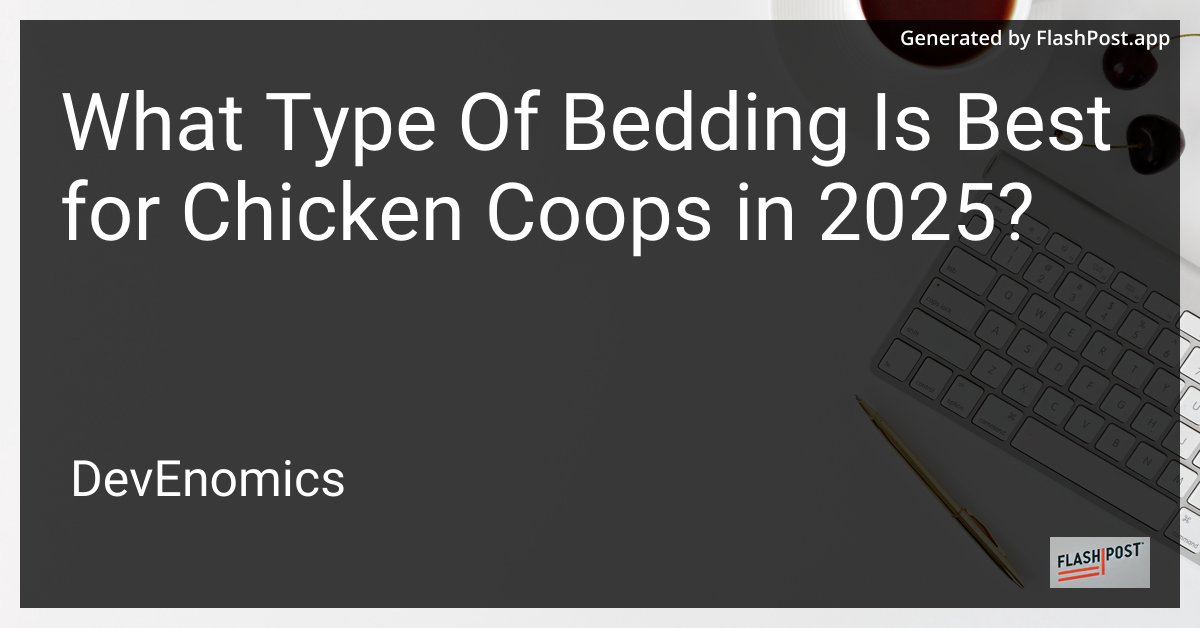
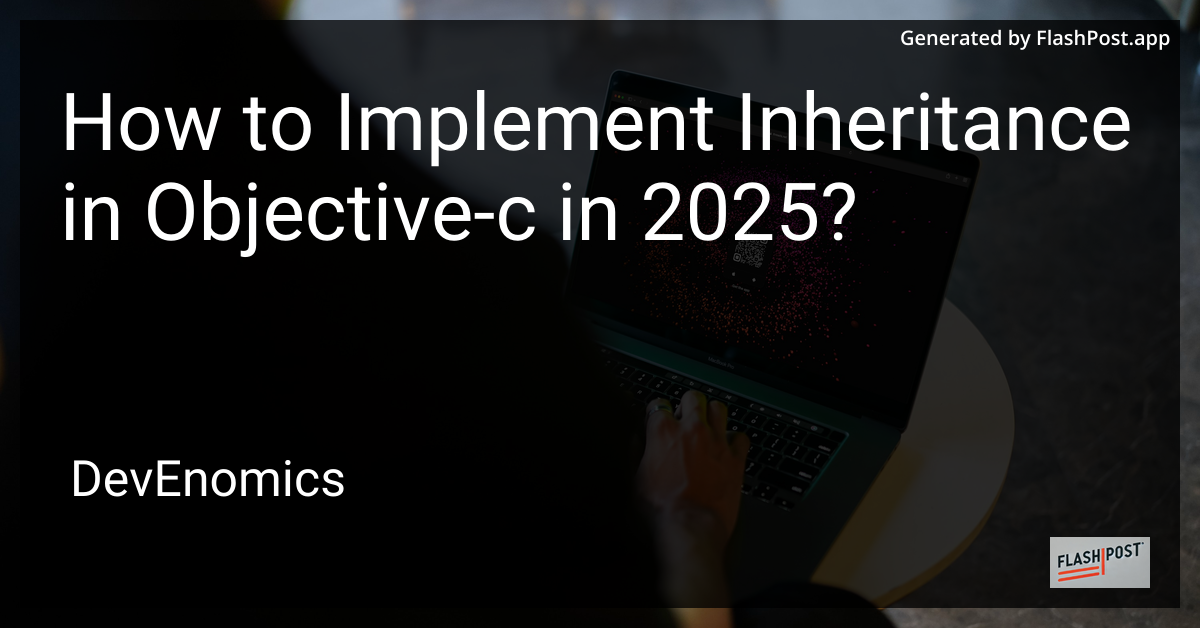
How to Implement Inheritance in Objective-c in 2025?
Objective-C remains a powerful programming language predominantly used for iOS and macOS applications. A primary feature that strengthens its utility is inheritance. Despite newer languages like Swift, Objective-C is still relevant due to its robustness and versatility, especially in legacy systems. In this guide, we’ll explore implementing inheritance in Objective-C, covering the core concepts and best practices suitable for 2025.
Understanding Inheritance in Objective-C
Inheritance is a fundamental object-oriented programming concept where a new class (subclass) inherits the properties and behavior (methods) of another class (superclass). This establishes a relationship that provides code reusability and a hierarchical class structure.
Why Use Inheritance?
- Code Reusability: Share methods and properties across multiple classes to avoid redundancy.
- Logical Structure: Create a clear, hierarchical structure that mirrors real-world relationships.
- Enhanced Functionality: Subclasses can add or modify the features of the parent class.
Steps to Implement Inheritance
Step 1: Define the Superclass
First, create a base class that other classes will inherit from. This superclass contains the generalized methods and properties.
#import <Foundation/Foundation.h>
@interface Vehicle : NSObject
@property NSString *make;
@property NSString *model;
- (void)startEngine;
- (void)stopEngine;
@end
@implementation Vehicle
- (void)startEngine {
NSLog(@"Engine started");
}
- (void)stopEngine {
NSLog(@"Engine stopped");
}
@end
Step 2: Create a Subclass
The subclass inherits from the superclass and can override or extend its capabilities.
#import "Vehicle.h"
@interface Car : Vehicle
@property int numberOfDoors;
- (void)openTrunk;
@end
@implementation Car
- (void)openTrunk {
NSLog(@"Trunk opened");
}
// Optionally, override a superclass method
- (void)startEngine {
[super startEngine];
NSLog(@"Car engine started with safety checks");
}
@end
Best Practices for 2025
- Use Modern Syntax: Leverage property and method declarations to keep your code clean and concise.
- Utilize ARC (Automatic Reference Counting): Ensure memory management is efficient and less error-prone.
- Integration with Swift: If combining with Swift, ensure compatibility by properly marking methods and properties with
@objc
.
Additional Resources
- For those seeking deeper learning, take advantage of current Objective-C book discounts.
- To ensure your codebase is error-free and maintainable, explore Objective-C code analysis tools.
- If your project involves advanced functionality like blocks, learn how to document them properly with Objective-C blocks documentation.
Conclusion
Implementing inheritance in Objective-C offers significant benefits, including cleaner code and a more logical architecture. Whether developing new applications or maintaining existing systems, mastering inheritance will enhance your Objective-C skills and contribute to project success well into 2025 and beyond.
Remember to keep your tools and knowledge up-to-date by exploring related resources and staying informed on best practices. Happy coding!
This article provides a comprehensive guide to implementing inheritance in Objective-C, optimized for search engines with relevant keywords and structured formatting. The included links offer readers further resources to deepen their knowledge and stay updated.