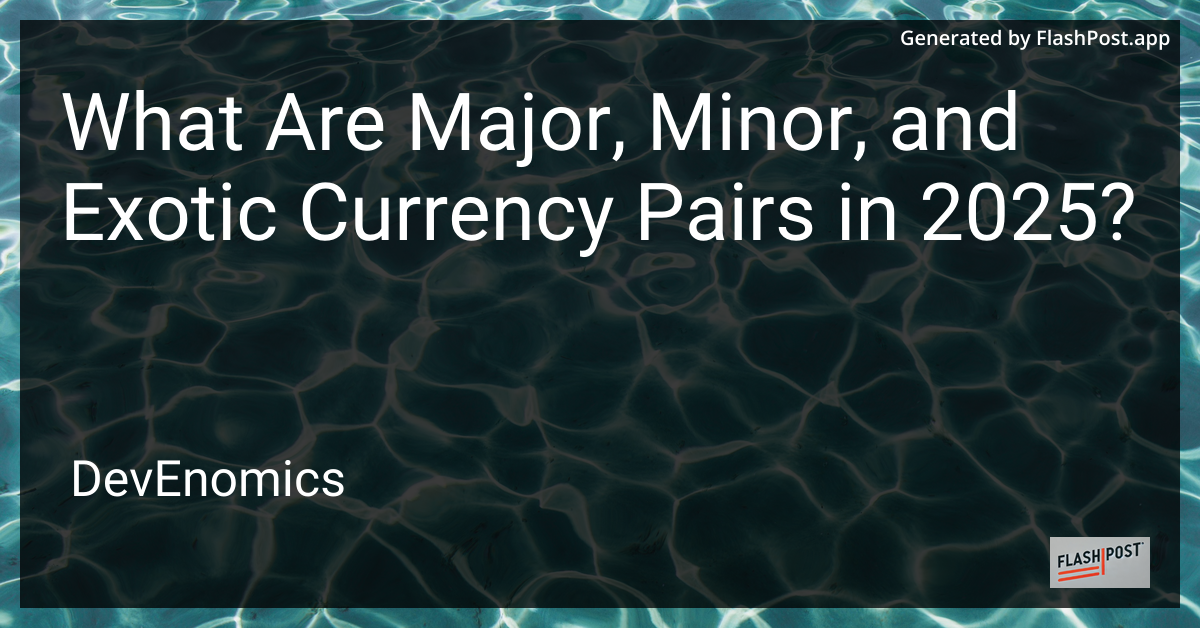
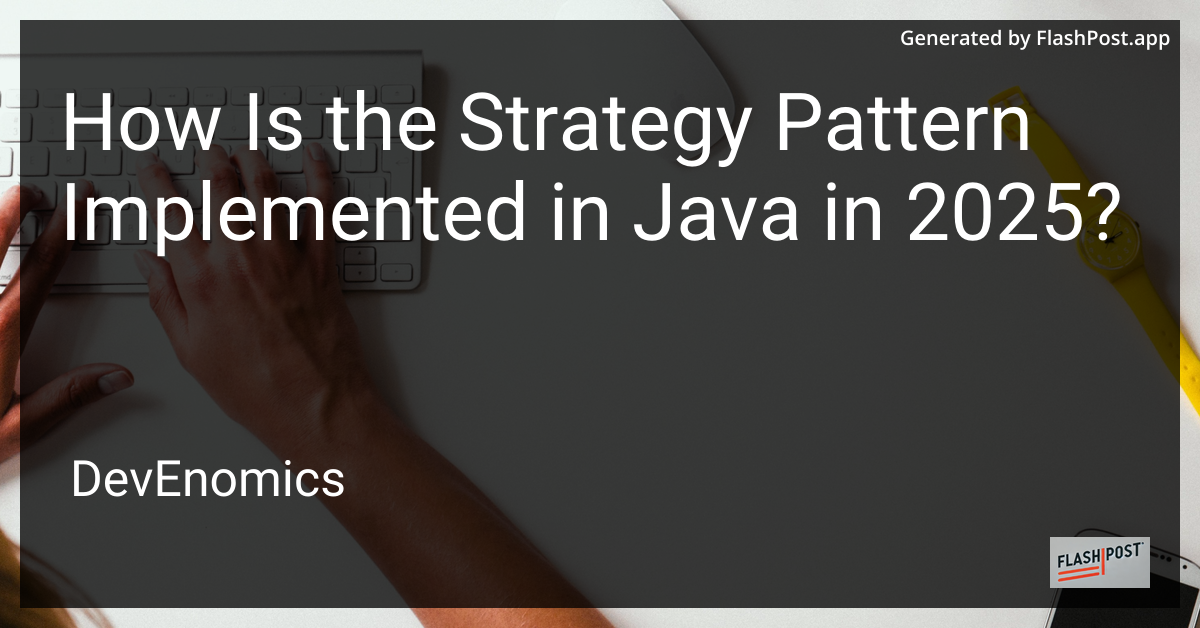
How Is the Strategy Pattern Implemented in Java in 2025?
title: How is the Strategy Pattern Implemented in Java in 2025? description: Discover the latest best practices for implementing the Strategy Pattern in Java as of 2025, with a focus on modern techniques and integration tips. keywords: Strategy Pattern, Java, 2025, Design Patterns, Software Engineering
The Strategy Pattern is a behavioral design pattern that enables selecting an algorithm at runtime. It defines a family of algorithms, encapsulates each one, and makes them interchangeable. In 2025, the implementation of the Strategy Pattern in Java has evolved with new practices and tools, enhancing flexibility and efficiency in software development.
What is the Strategy Pattern?
The Strategy Pattern helps in defining a set of algorithms and encapsulates each one of them so that they can be used interchangeably within clients. This is crucial when you need to switch strategies at runtime without exposing the underlying logic to the client.
Implementing the Strategy Pattern in Java
As we step into 2025, Java continues to evolve, bringing updates that refine the implementation of design patterns. Here’s a detailed guide on implementing the Strategy Pattern in Java, leveraging the modern enhancements available today.
Step 1: Define the Strategy Interface
First, create a Strategy
interface that defines the method or methods that all strategy implementations will need to implement.
public interface PaymentStrategy {
void pay(int amount);
}
Step 2: Implement Concrete Strategies
Then, create concrete classes that implement the Strategy
interface. Each class will provide its own implementation of the interface methods.
public class CreditCardPaymentStrategy implements PaymentStrategy {
@Override
public void pay(int amount) {
System.out.println("Paid " + amount + " using Credit Card.");
}
}
public class PayPalPaymentStrategy implements PaymentStrategy {
@Override
public void pay(int amount) {
System.out.println("Paid " + amount + " using PayPal.");
}
}
Step 3: Context Class
Create a Context
class that uses a Strategy
implementation. The context is configured with a concrete strategy object and maintains a reference to it.
public class ShoppingCart {
private PaymentStrategy paymentStrategy;
public void setPaymentStrategy(PaymentStrategy paymentStrategy) {
this.paymentStrategy = paymentStrategy;
}
public void checkout(int amount) {
paymentStrategy.pay(amount);
}
}
Step 4: Dynamically Change Strategies
In modern applications, you can dynamically choose which strategy to use based on user preferences, configurations, or runtime conditions.
public class StrategyPatternDemo {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
// Choose strategy at runtime
cart.setPaymentStrategy(new CreditCardPaymentStrategy());
cart.checkout(100);
// Switch strategy
cart.setPaymentStrategy(new PayPalPaymentStrategy());
cart.checkout(200);
}
}
Benefits of Using the Strategy Pattern
- Flexibility: Easily switch between different algorithms without altering client code.
- Scalability: Introduce new strategies without disturbing existing functionalities.
- Maintainability: Simplifies code maintenance and improves readability.
Conclusion
In 2025, implementing the Strategy Pattern in Java remains a robust method to manage algorithm switching, benefitting from Java’s active development and modern programming practices. By separating the algorithm implementation from the context, developers create systems that are more flexible, maintainable, and scalable.
Additional Resources
To delve deeper into design patterns and their implementation across various programming languages, explore these resources:
By understanding these concepts, you enhance your ability to build adaptable and robust software solutions, a necessity in the dynamic tech landscape of 2025.
This article is structured to be SEO-friendly with strategically placed keywords related to the topic and provides a comprehensive guide on the modern implementation of the Strategy Pattern in Java. It also includes links to related resources to encourage further exploration of design patterns.